آموزش نحوه ارسال و دریافت شی از تابع در C++ (به زبان کاملا ساده)
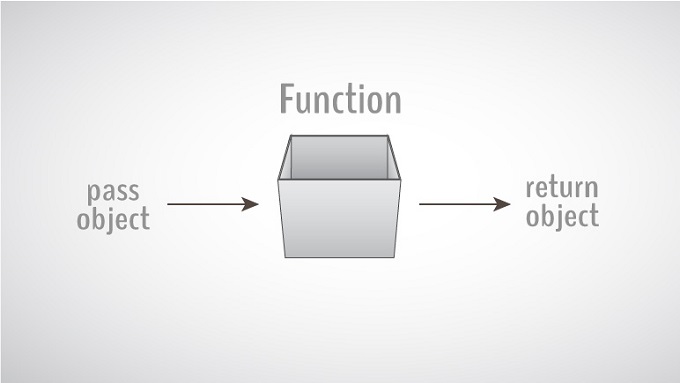
در این آموزش، یاد خواهید گرفت که اشیاء را به تابع ارسال و دریافت کنید.
در برنامه نویسی C++، اشیا به شیوه ای مشابه با ساختار ها (struct) به تابع ارسال می شوند.
چگونه می توان اشیاء را به تابع انتقال داد؟
مثال ۱ : انتقال اشیا به تابع
برنامه زیر دو عدد پیچیده(شی) را با ارسال آن ها به تابع جمع می کند.
- #include <iostream>
- using namespace std;
- class Complex
- {
- private:
- int real;
- int imag;
- public:
- Complex(): real(0), imag(0) { }
- void readData()
- {
- cout << “Enter real and imaginary number respectively:”<<endl;
- cin >> real >> imag;
- }
- void addComplexNumbers(Complex comp1, Complex comp2)
- {
- // real represents the real data of object c3 because this function is called using code c3.add(c1,c2);
- real=comp1.real+comp2.real;
- // imag represents the imag data of object c3 because this function is called using code c3.add(c1,c2);
- imag=comp1.imag+comp2.imag;
- }
- void displaySum()
- {
- cout << “Sum = ” << real<< “+” << imag << “i”;
- }
- };
- int main()
- {
- Complex c1,c2,c3;
- c1.readData();
- c2.readData();
- c3.addComplexNumbers(c1, c2);
- c3.displaySum();
- return 0;
- }
خروجی
Enter real and imaginary number respectively:
۲
۴
Enter real and imaginary number respectively:
-۳
۴
Sum = -1+8i
چگونه از تابع شی برگردانیم؟
در برنامه نویسی C++، اشیا همانند ساختار ها (struct) بازگردانده می شوند.
مثال ۲ : ارسال و بازگشت شی از تابع
در این برنامه، مجموع اعداد پیچیده (شی) به تابع ()main باز گردانده و نمایش داده می شود.
- #include <iostream>
- using namespace std;
- class Complex
- {
- private:
- int real;
- int imag;
- public:
- Complex(): real(0), imag(0) { }
- void readData()
- {
- cout << “Enter real and imaginary number respectively:”<<endl;
- cin >> real >> imag;
- }
- Complex addComplexNumbers(Complex comp2)
- {
- Complex temp;
- // real represents the real data of object c3 because this function is called using code c3.add(c1,c2);
- temp.real = real+comp2.real;
- // imag represents the imag data of object c3 because this function is called using code c3.add(c1,c2);
- temp.imag = imag+comp2.imag;
- return temp;
- }
- void displayData()
- {
- cout << “Sum = ” << real << “+” << imag << “i”;
- }
- };
- int main()
- {
- Complex c1, c2, c3;
- c1.readData();
- c2.readData();
- c3 = c1.addComplexNumbers(c2);
- c3.displayData();
- return 0;
- }